The first step
After we had established the basic "Framework" in Step 00 we now add a little dynamic into the application.
Features
In this project we let a recangle with some text bounce around on the screen always staying inside the screen.
Upcoming
As a next step we could build the "Windows Starfield Screensaver" now to introduce 3D Vectors, and Entities.
Or we take a step into input handling and allow the user to spawn custom texts
and add controls to the text.
Details
For this Project i needed a few Classes that i can reuse in my library again. For the the handling of collision and calculation of the edge-bounce i abstracted the logic into Vector2D and Rectangles and added some usefull functions to them.
In the end in the GameModel i only needed to call a few lines of code to realize the bouncing.
First i had created a rectangle that represented the area that the text is not allowed to leave. This is done by taking the entire screen area and "inset" it by the size of the text-box.
Meaning the borders of the screen recangle get offset inwards, such that the text-box fits exactly between the new border and the old border on all sides (top, right, bottom, left).
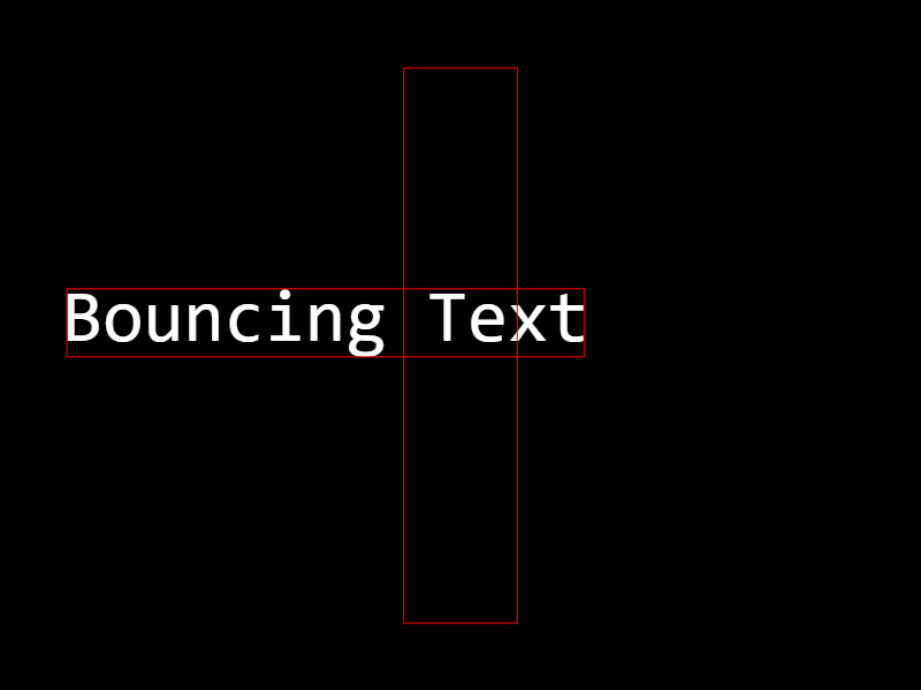
Now the idea is that whenever the text-box is not touching this inner rectangle anymore it is touching a border.
For this i have created the "distance" function on the Rect class, which calculates the horizontal and vertical distance between two rectangles.
If they overlap, the distance is 0.
public update(delta_seconds: number) { // update text position this.text_box.move(this.text_velocity.cpy().mul(delta_seconds / 1000)); // if the distance to this inner box is greater than 0, the text box is outside the screen box const distance = this.screen_box_inset.distance(this.text_box); if (Math.abs(distance.x) > 0) { this.text_velocity.x = Math.abs(this.text_velocity.x) * ((distance.x > 0) ? -1 : 1); this.text_box.center.x -= distance.x * 2; } if (Math.abs(distance.y) > 0) { this.text_velocity.y = Math.abs(this.text_velocity.y) * ((distance.y > 0) ? -1 : 1); this.text_box.center.y -= distance.y * 2; } }
Now if there is a distance that is not 0, I know that i have to handle a bounce, by changing the velocity in the opposite direction, where the rectangle has overshoot also moving the text in the opposite direction of the overshoot.
This would be the position the rectangle would have if i would more frequent updates of the "physics" simulation.
Comments